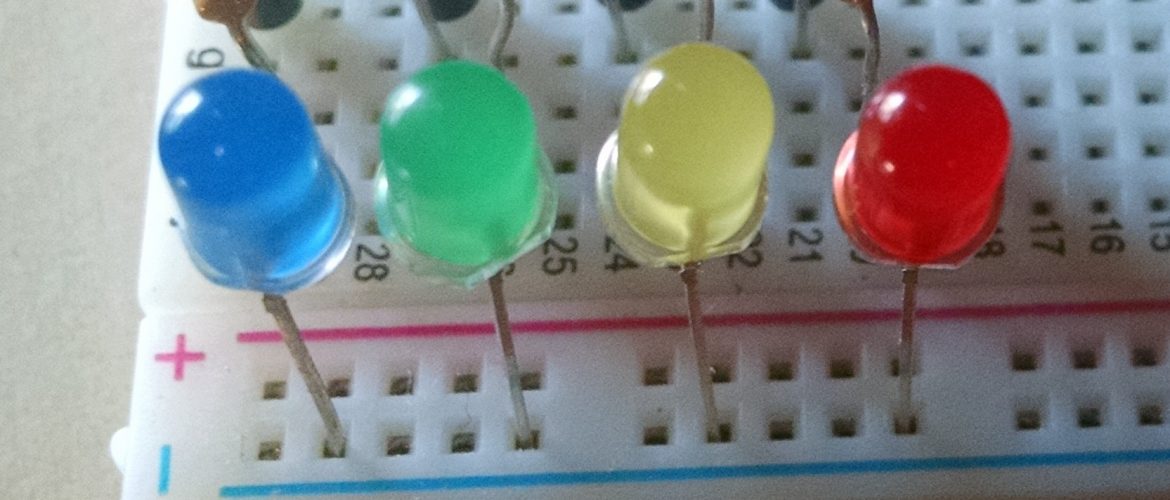
Emotions and Twitter
Author: Aimee Albro - Account Director
The most recent project I’ve been working on involves a Raspberry Pi, the Twitter API, and some LEDs in a breadboard. Jeffrey pitched me the idea of monitoring the “global mood” of the Internet through tracking tweets. So, I got to work setting up the Raspberry Pi that was lying around the office and put it to good use.
For this project I made my first foray into the world of Python, specifically Python3.4. To simplify things, I found a library to connect to the Twitter API with, Tweepy. Tweepy makes it simple to connect to a streaming endpoint and search for specific words or phrases. I searched for four specific hashtags: happy, sad, angry and jealous.
The script itself is run from the Terminal in Linux, and this is the main body of it. There is a StdOutListener
class that I have defined and will discuss later in the post. The Twitter API uses OAuth for authentication, requiring a Twitter application to be created with a Consumer Key, Consumer Secret, Access Token and Access Token Secret. Tweepy uses these to authenticate with Twitter and create a Streaming connection. The stream.filter()
is what is used to specify which tweets you want to receive. Once the Stream is started, we use Ctrl + C to stop the script. Occasionally the stream will time out, which is why the while-true
loop is being used. If any exception other than KeyboardInterrupt
is thrown, then the stream is restarted.
l = StdOutListener()
auth = OAuthHandler(consumer_key, consumer_secret)
auth.set_access_token(access_token, access_token_secret)
while True:
try: #This handles connection to Twitter Streaming API
stream = Stream(auth, l)#, timeout=90)
stream.filter(track=['#happy','#sad','#angry','#jealous'])
except KeyboardInterrupt:
GPIO.cleanup()
quit()
except:
continue
Now, let’s take a look at the StdOutListener
class that handles the tweets being received. The StdOutListener
is a subclass of the StreamListener
class in the Tweepy library. The Twitter API returns JSON data with all relevant information about the given tweet. The only thing we are interested in is the body of the tweet itself, so we extract that and store it in the text
variable. There are two global variables: PRINT_TWEETS
and PRINT_MODE
, that print the body of the tweet or the specific keyword found in that tweet, respectively. We then search for each specific keyword in that tweet and light up a corresponding LED. I only included the search for #happy, but the others follow the same pattern.
class StdOutListener(StreamListener):
def on_data(self, data):
jsonData = json.loads(data)
text = jsonData["text"]
if PRINT_TWEETS:
print(text)
if (text.find("#happy") >= 0):
if PRINT_MODE:
print ("Happy")
GPIO.output(YELLOW_LED, True)
sleep(0.5)
GPIO.output(YELLOW_LED, False)
.
.
.
.
def on_error(self, status):
print("Error: "+str(status))
Each keyword corresponds with a certain color: yellow for happy, blue for sad, green for jealous, and red for angry. They are in a breadboard, which uses a Pi Cobbler to connect the GPIO pins of the Raspberry Pi. This way, power can be output to a specific GPIO numbered pin, to light up one of the colored LEDs.
You can see a short video of it in action here:
Want to learn more about how Hark can help?